Add Error Monitoring to an Express.js App with Rollbar
Stay on top of errors in your Node.js apps to find and fix bugs faster.
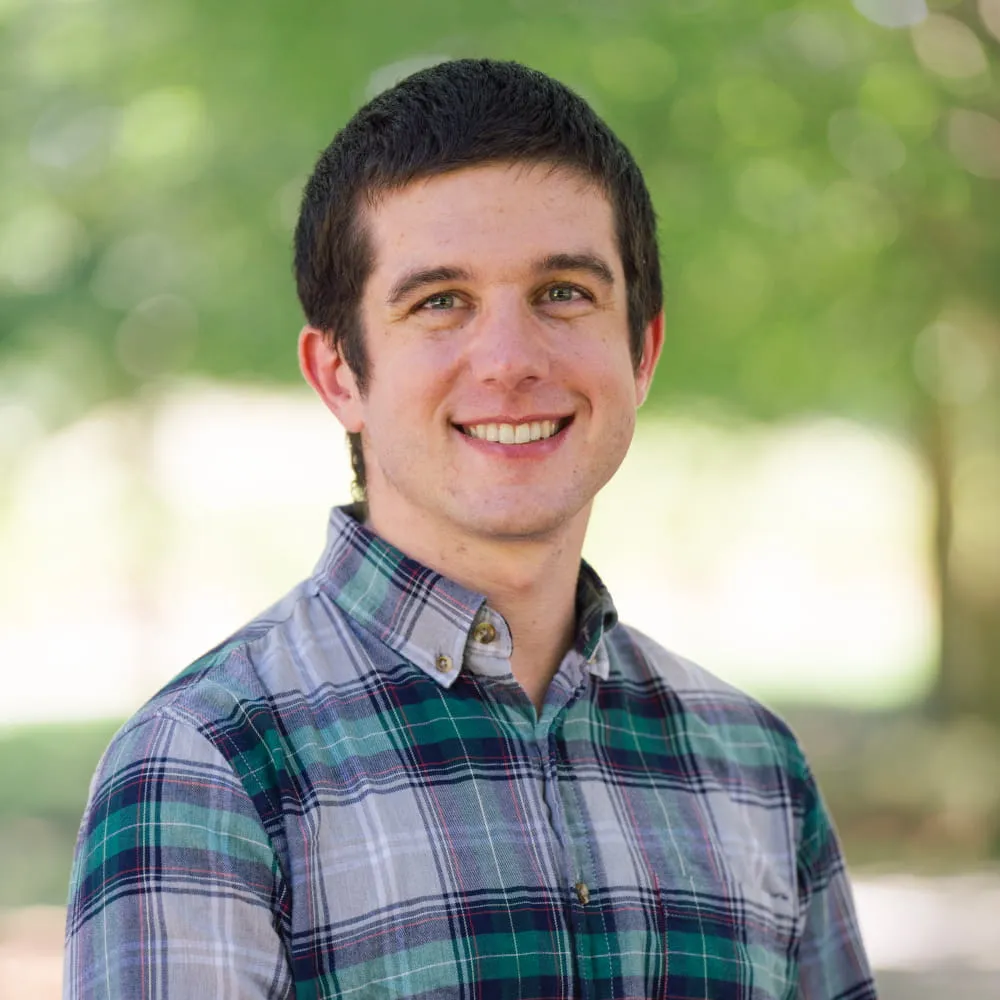
Sam Magura
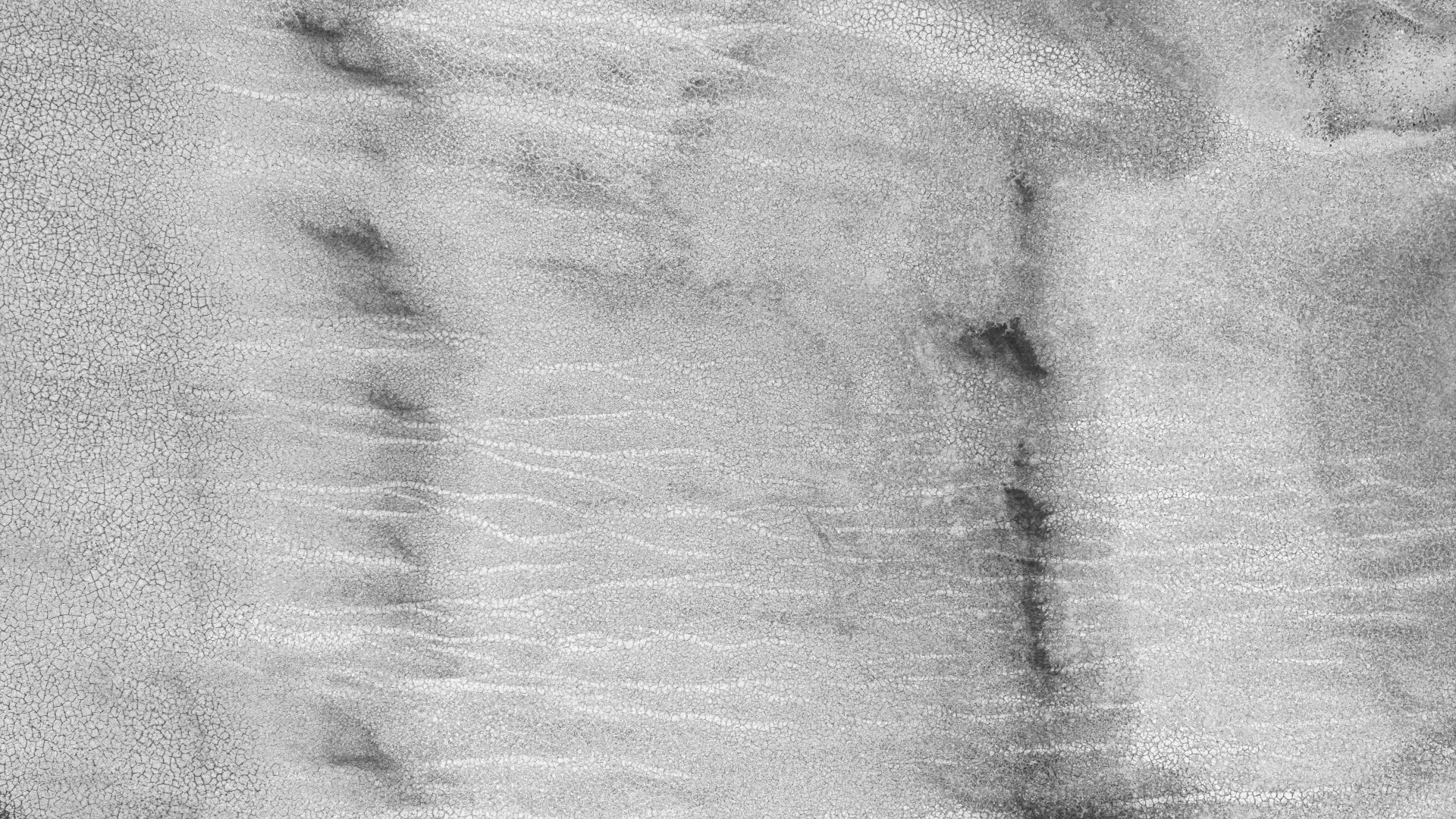
Without proper error monitoring in your application, you won't know about a bug until a user reports it. At that point, the bug has likely affected hundreds of users. It's much more effective to implement an error monitoring solution in your app so that you receive a notification as soon as an error occurs.
Rollbar is one of the leading error monitoring platforms, with SDKs for almost every server and client platform. When your app hits an error, the error details get sent to Rollbar. Rollbar then displays the error in its user-friendly dashboard and sends you a notification. The dashboard allows you to view useful statistics, like the number of affected users, and how the number of occurrences of the error has changed over time.
In this article, we'll walk through integrating the Rollbar Node.js SDK into an Express-based web API. The Zero secrets manager will be used to securely store the Rollbar access token.
🔗 The full code for this example is available in the zerosecrets/examples GitHub repository.
Secure your secrets conveniently
Zero is a modern secrets manager built with usability at its core. Reliable and secure, it saves time and effort.
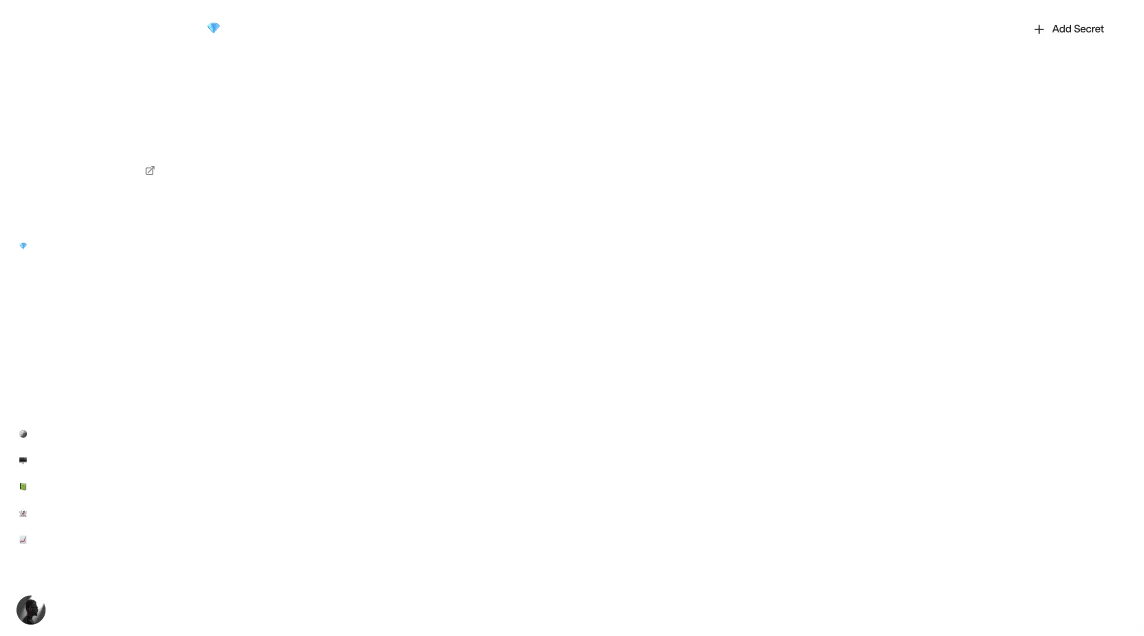
Signing up for Rollbar
Click here to sign up for Rollbar. When prompted, create a new project and select Node.js for the technology. This will display a "getting started" code snippet that includes your Rollbar access token.
Let's store the access token in Zero for safe keeping. Log in to Zero and create a new project, saving the token to a file on your local PC. Then, add a new secret and paste in the Rollbar access token:
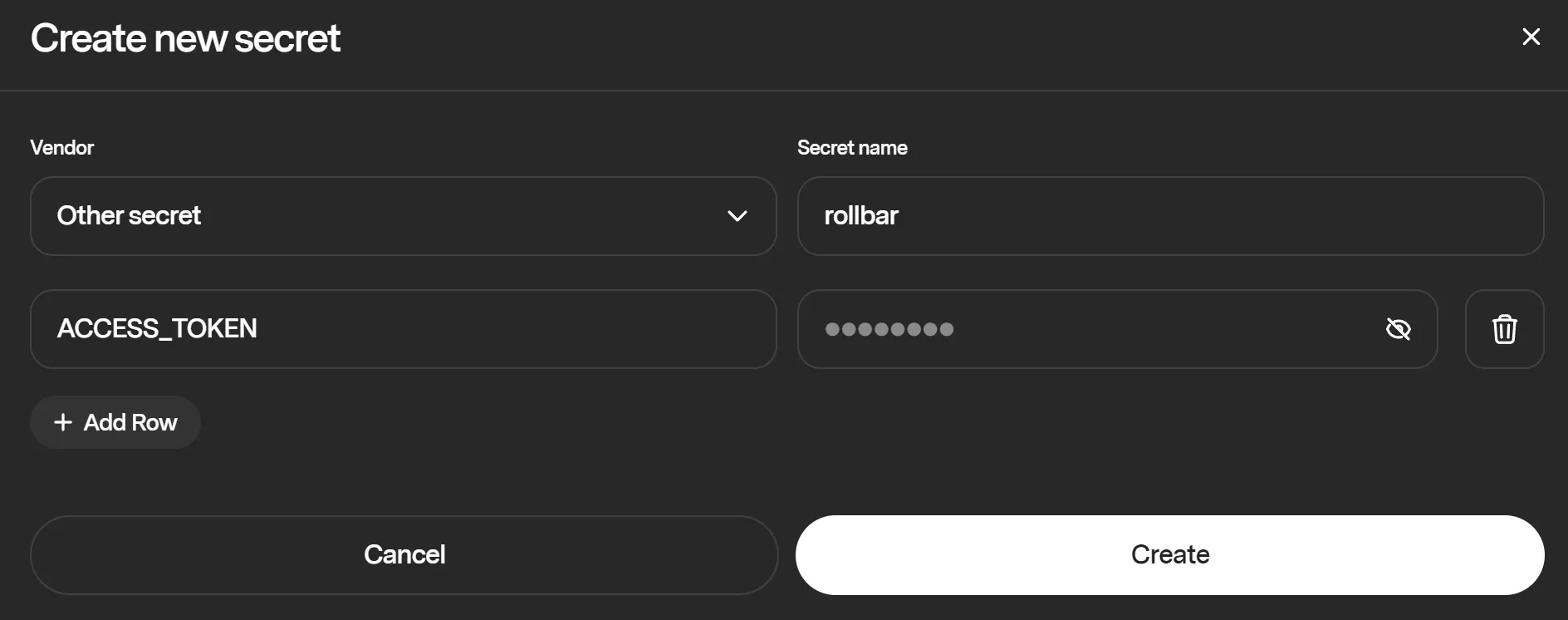
Bootstrapping an Express App
Now let's start coding. First, we'll need a basic Express web application using TypeScript. The exact steps for setting up this kind of app can be found in my post on integrating with the OpenAI API .
In addition to installing the Zero TypeScript SDK , you'll also want to install the rollbar
npm package:
npm install rollbar @zerosecrets/zero
Now, let's create a basic web API. We'll have two methods, one which returns a successful response, and one which throws an error. The Express code for this looks like this:
import express from 'express'
const PORT = 3000
const app = express()
app.get('/', async (req, res) => {
res.send(JSON.stringify('success'))
})
app.get('/error', () => {
throw new Error('test error')
})
app.listen(PORT, () => {
console.log(`Server listening on port ${PORT}...`)
})
You can call the API by running npm start
and then navigating to localhost:3000
or localhost:3000/error
in your web browser.
Initializing the Rollbar SDK
The Rollbar SDK can be initialized using the standard pattern for initializing a third party API client with Zero. Create a file called getRollbar.ts
and add the following code:
import {zero} from '@zerosecrets/zero'
import Rollbar from 'rollbar'
let rollbar: Rollbar | undefined
export async function getRollbar(): Promise<Rollbar> {
// Reuse the same Rollbar client if one has already been created, so that we
// don't call Zero on every request
if (rollbar) {
return rollbar
}
if (!process.env.ZERO_TOKEN) {
throw new Error('Did you forget to set the ZERO_TOKEN environment variable?')
}
const secrets = await zero({
token: process.env.ZERO_TOKEN,
pick: ['rollbar'],
}).fetch()
if (!secrets.rollbar) {
throw new Error('Did not receive an API key for Rollbar.')
}
rollbar = new Rollbar({
accessToken: secrets.rollbar.access_token,
captureUncaught: true,
captureUnhandledRejections: true,
})
return rollbar
}
Adding Basic Logging
In main.ts
, we should set up the Rollbar SDK as soon as possible so that any uncaught errors that occur during startup get captured. So add the line
const rollbar = await getRollbar()
directly under the import
s section of your file.
Rollbar can be used to log messages of any kind, not only errors. Let's try that out by logging when the app starts up:
app.listen(PORT, () => {
console.log(`Server listening on port ${PORT}...`)
rollbar.log('App started up')
})
Adding Error Handling
We also want to capture the error that occurs when the /error
API method is called. To do this, we could wrap the API handler in a try-catch
, but this would get very tedious if we had to do it for every API method in the entire app. It's much more convenient to use an Express error handler .
An Express error handler is a special kind of middleware that accepts an Error
object as its first argument, in addition to the normal req
, res
, and next
arguments that are passed to middlewares. The important thing when defining an error handler is: The app.use
call that adds the error handler must occur after every other middleware and request handler has been registered!
So, this code should be placed directly above the app.listen
call, below all of the app.get
, app.post
, etc. calls.
app.use((err: Error, req: Request, res: Response, next: NextFunction) => {
rollbar.error(err)
next(err)
})
Now, errors thrown by any API method will be sent to Rollbar!
To try it out, restart the app and hit localhost:3000/error
in your browser. Now check the "Items" tab in the Rollbar dashboard, and see you'll see both the error and the "App started up" message:
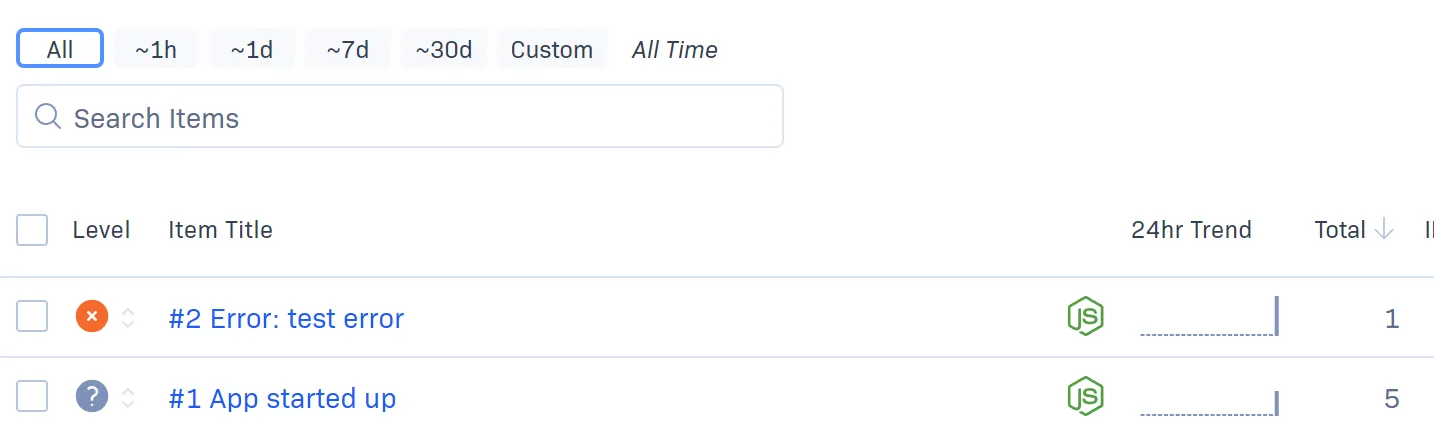
Next Steps
This post showed you how to quickly integrate Rollbar into a Node.js web server, using Zero to securely manage the Rollbar access token. To take this a step further, you can also set up Rollbar on the client-side to catch errors in your web or mobile frontend. Zero is not necessary when using the Rollbar client-side SDKs since the token used there is publicly-visible, not a secret.
Other articles
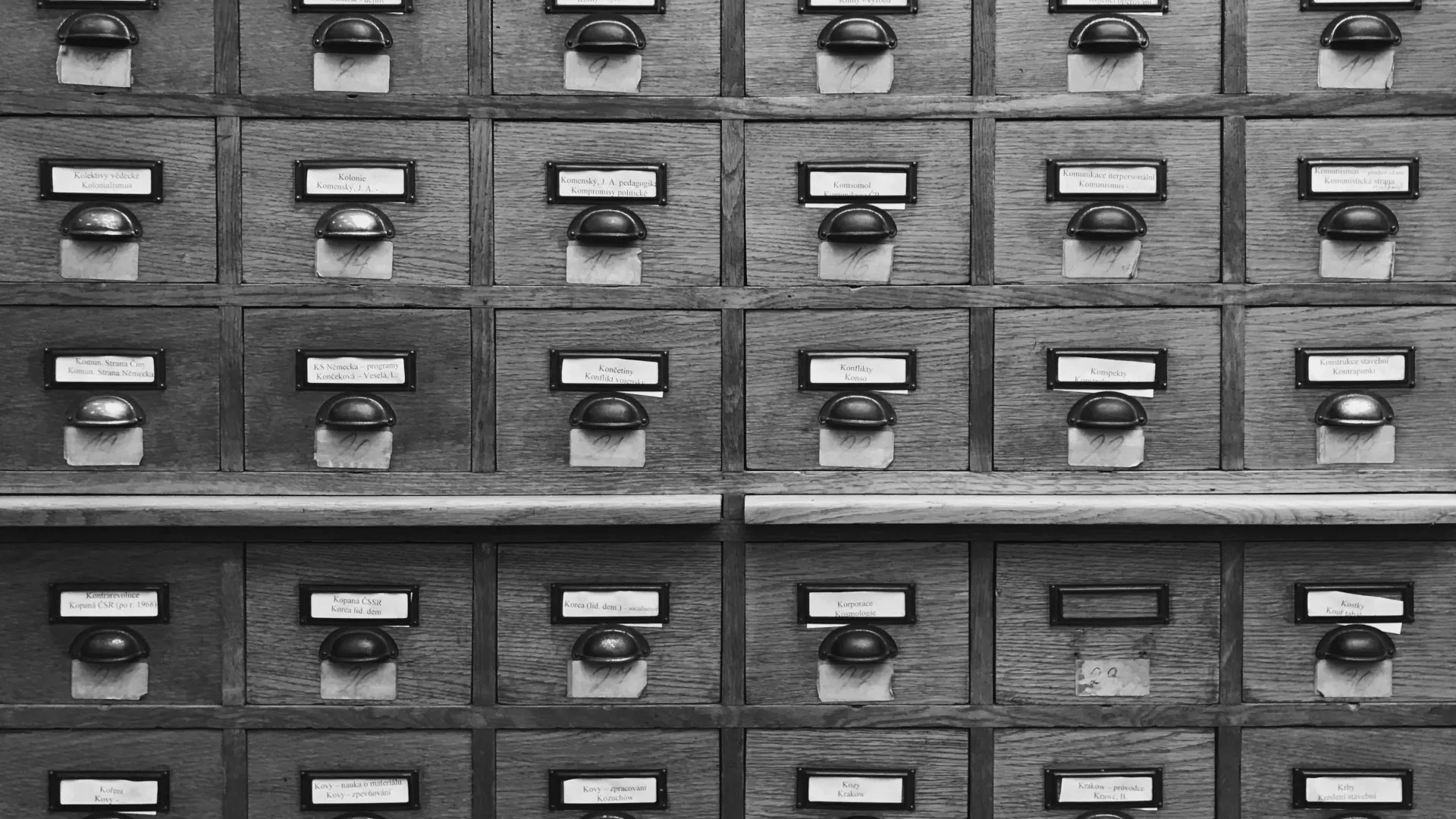
Using Notion as a Human-Readable Database
Capture form submissions from your web app and store them where your team works.
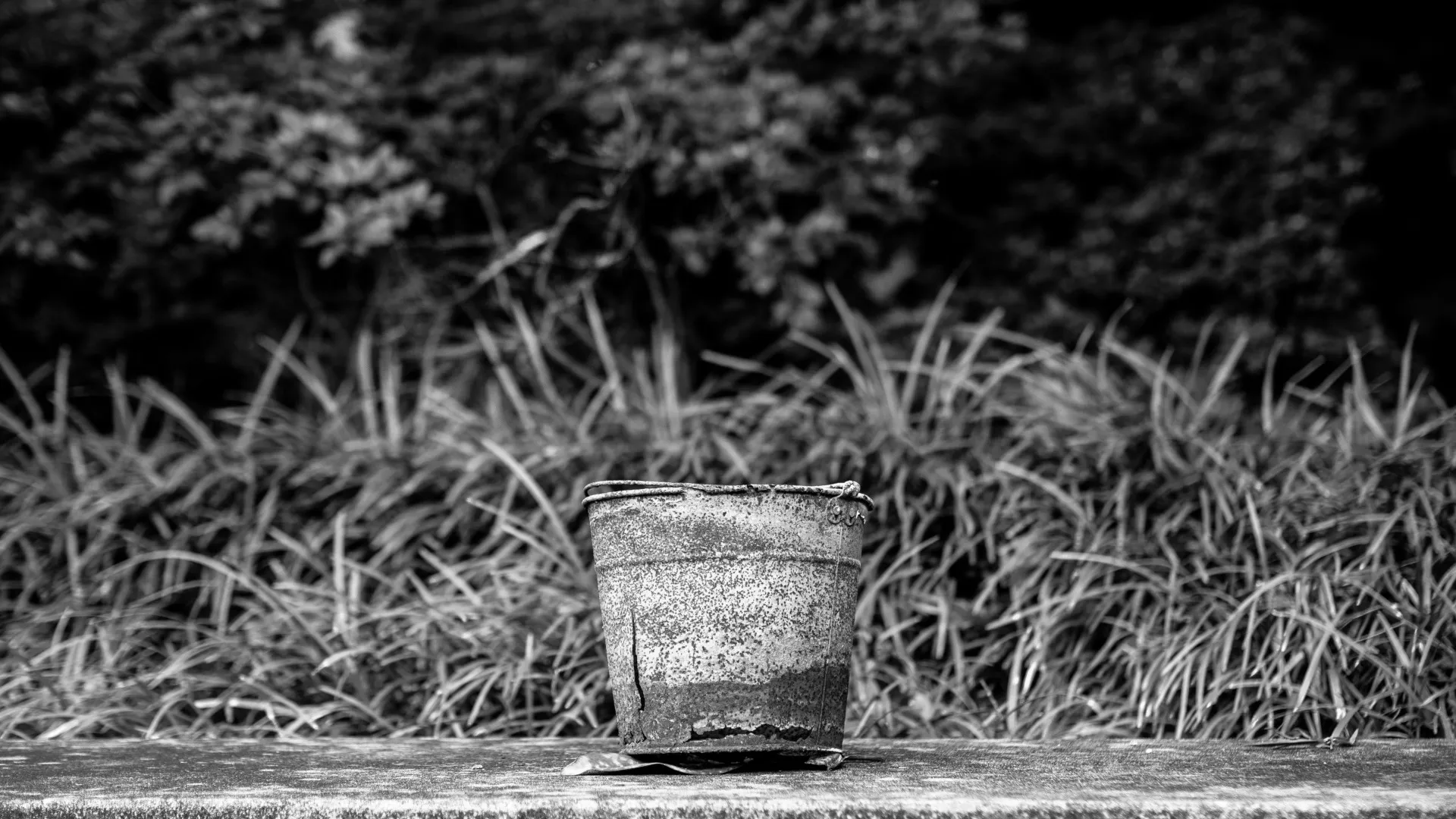
Managing Files in Amazon S3 from Node.js
Adding and removing files from S3 is a breeze with the AWS JavaScript SDK.
Secure your secrets
Zero is a modern secrets manager built with usability at its core. Reliable and secure, it saves time and effort.